Generics
1 | function hello(message: any) : any { |
1 | function helloGeneric<T> (message:T) :T { // 함수의 안에서 T의 타입을 기억하게 된다 |

1 | function helloGeneric<T> (message:T) :T { // 함수의 안에서 T의 타입을 기억하게 된다 |
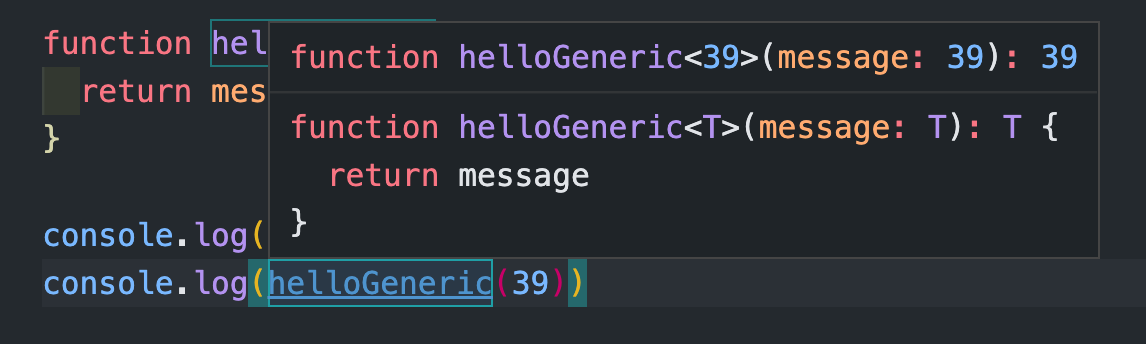
1 | function helloGeneric<T> (message:T) :T { // 함수의 안에서 T의 타입을 기억하게 된다 |
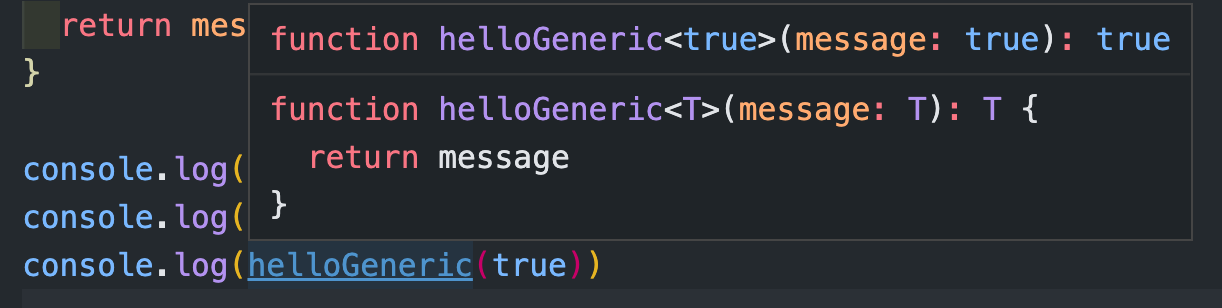
Generics Basic
1 | function helloBasic<T>(message: T): T { |
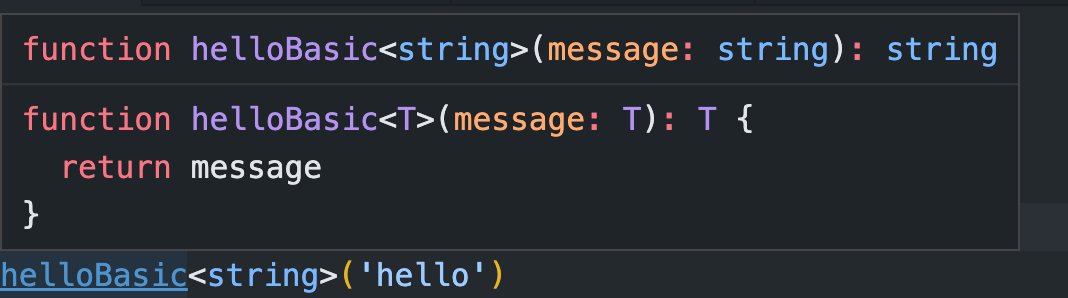

Generics Array & Tuple
1 | function helloArray<T>(message: T[]): T { |
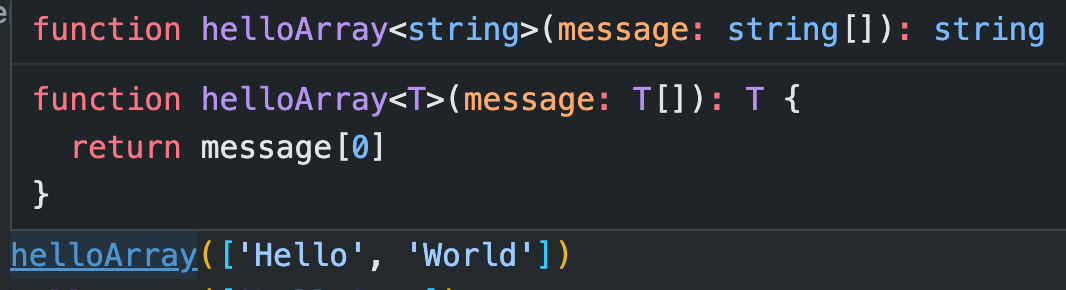
string으로 추론

string과 number로 추론

사용할 수 있는 메서드가 제한적이다
1 | function helloTuple<T, K>(message: [T, K]): T { |

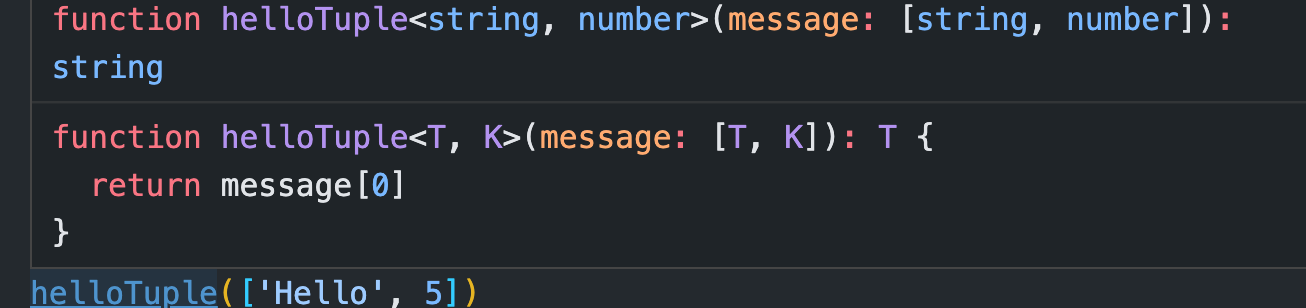
Generics Function
1 | // type alias |
Generics Class
1 | class Person<T> { |
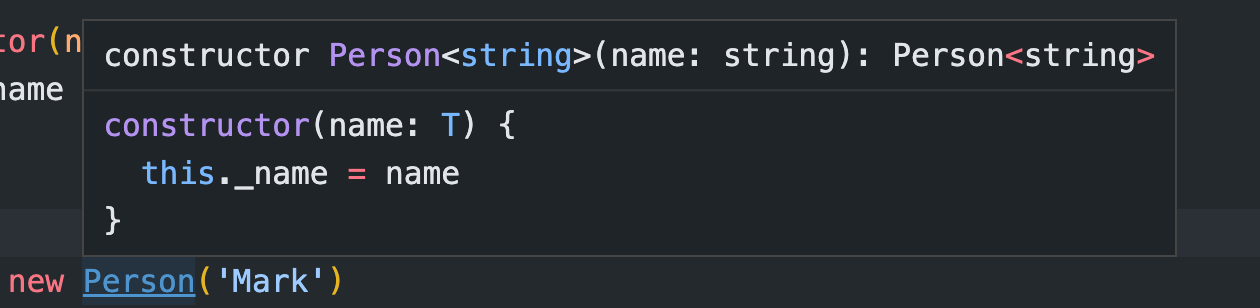
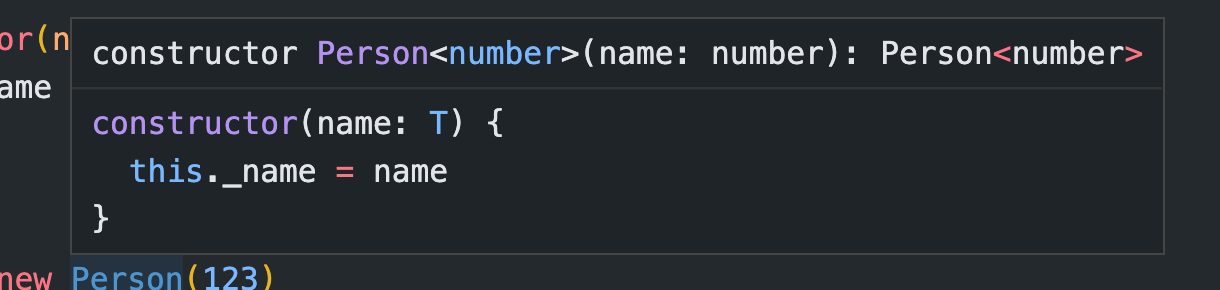
1 | class Person<T, K> { |

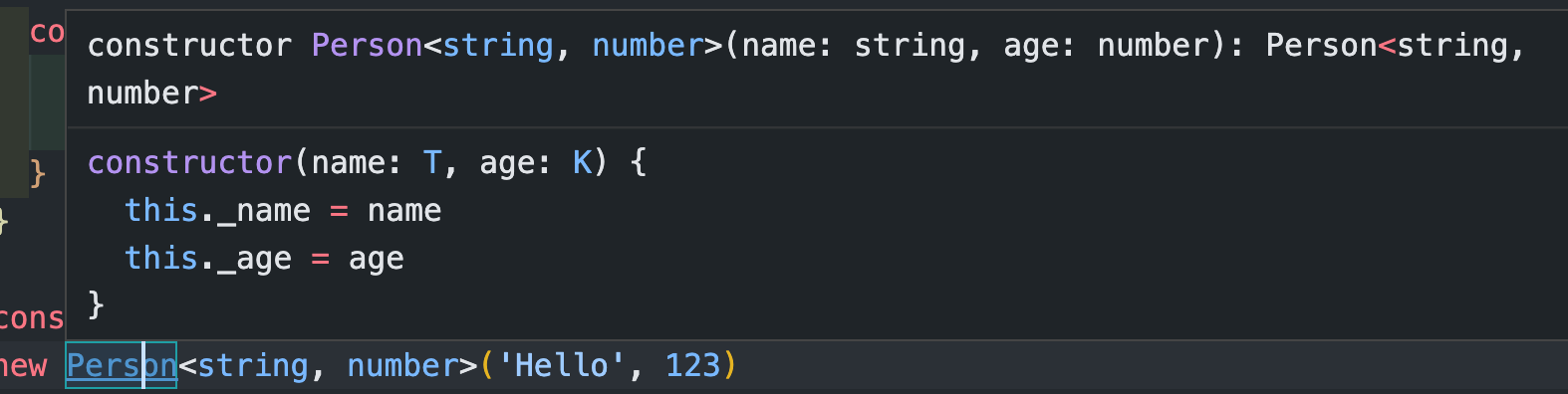
Generics with extends
1 | class PersonExtends<T extends string | number> { |

keyof & type lookup system
1 | interface IPerson { |