interface
1 | interface Person1 { |
optional property (1)
1 | interface Person2 { |
optional property (2)
1 | interface Person3 { |
function in interface
1 | interface Person4 { |
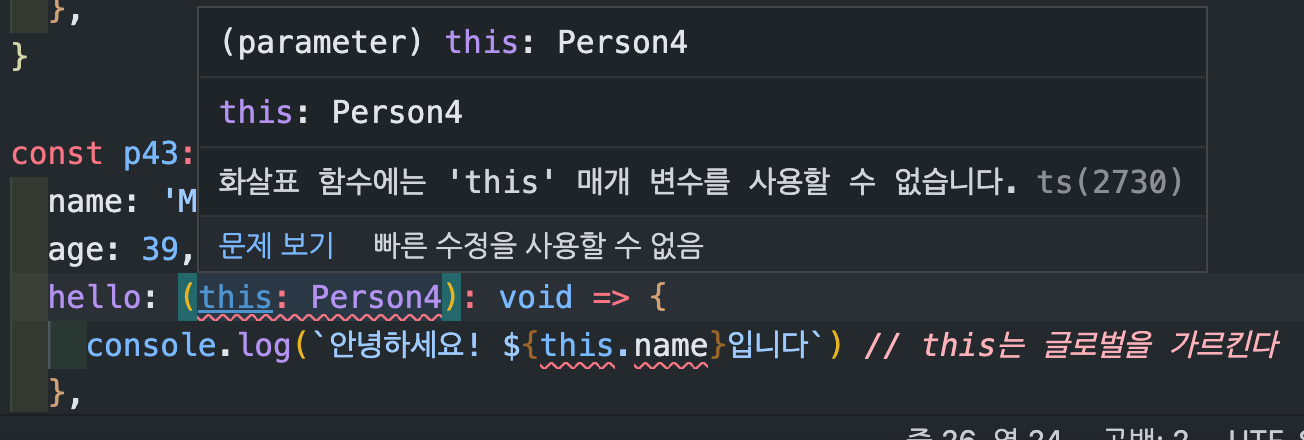
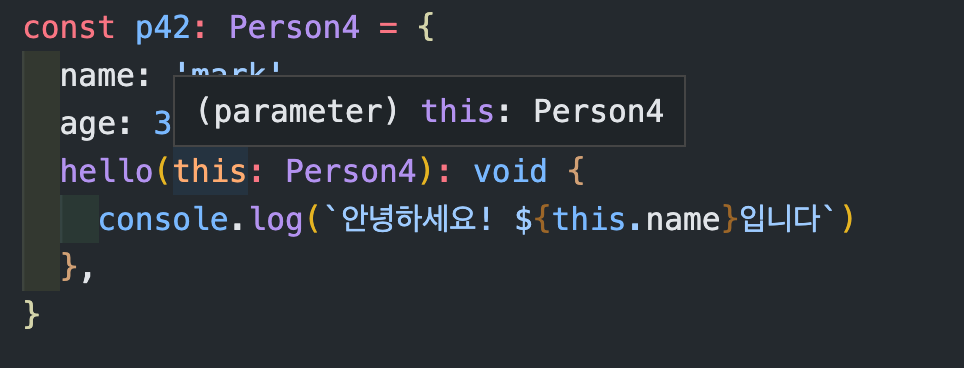
1 | interface Person1 { |
1 | interface Person2 { |
1 | interface Person3 { |
1 | interface Person4 { |