컴포넌트 추출
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43
| function formatDate(date) { return date.toLocaleDateString(); }
function Comment(props) { return ( <div className="Comment"> <div className="UserInfo"> <img className="Avatar" src={props.author.avatarUrl} alt={props.author.name} /> <div className="UserInfo-name"> {props.author.name} </div> </div> <div className="Comment-text"> {props.text} </div> <div className="Comment-date"> {formatDate(props.date)} </div> </div> ); }
const comment = { date: new Date(), text: 'I hope you enjoy learning React!', author: { name: 'Hello Kitty', avatarUrl: 'http://placekitten.com/g/64/64' } };
const root = ReactDOM.createRoot(document.getElementById('root')); root.render( <Comment date={comment.date} text={comment.text} author={comment.author} /> );
|
- 위 컴포넌트는 구성요소들이 모두 중첩 구조로 이루어져 있어서 변경하기 어려울수 있다.
- 구성요소를 개별적으로 재사용하기도 힘들다
- 구성요소를 컴포넌트로 추출한다
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42
| import React from 'react' function formatDate(date) { return date.toLocaleDateString() } const Avatar = (props) => { return ( <img className='Avatar' src={props.user.avatarUrl} alt={props.user.name} /> ) } const UserInfo = (props) => { return ( <div className='UserInfo'> <Avatar user={props.user} /> <div className='UserInfo-name'>{props.user.name}</div> </div> ) } function Comment(props) { return ( <div className='Comment'> <UserInfo user={props.author} /> <div className='Comment-text'>{props.text}</div> <div className='Comment-date'>{formatDate(props.date)}</div> </div> ) }
const comment = { date: new Date(), text: 'I hope you enjoy learning React!', author: { name: 'Hello Kitty', avatarUrl: 'http://placekitten.com/g/64/64', }, }
export default function Extraction() { return ( <Comment date={comment.date} text={comment.text} author={comment.author} /> ) }
|
- Extraction 컴포넌트에서 Comment 컴포넌트 props로 date,text,author라는 이름으로 넘겨주고 있다
1 2 3 4 5 6 7 8 9 10
| const Comment = (props) => { console.log(props) return ( <div className='Comment'> <UserInfo user={props.author} /> <div className='Comment-text'>{props.text}</div> <div className='Comment-date'>{formatDate(props.date)}</div> </div> ) }
|
Comment 컴포넌트의 props를 콘솔로 찍었을때
Comment 컴포넌트에서 UserInfo 컴포넌트로 user라는 이름으로 {author}를 넘겨 주고 있다
1 2 3 4 5 6 7 8 9
| const UserInfo = (props) => { console.log(props) return ( <div className='UserInfo'> <Avatar user={props.user} /> <div className='UserInfo-name'>{props.user.name}</div> </div> ) }
|
UserInfo 컴포넌트의 props를 콘솔로 찍었을 때
UserInfo 컴포넌트에서 Avatar 컴포넌트로 user라는 이름으로 {props.user} = {author} 를 넘겨 주고 있다
1 2 3 4 5 6
| const Avatar = (props) => { console.log(props) return ( <img className='Avatar' src={props.user.avatarUrl} alt={props.user.name} /> ) }
|
Avatar 컴포넌트에서 props를 찍었을때
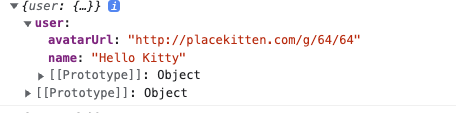