next js 중첩 레이아웃
프로젝트를 하던중 탭을 이용해 페이지 내에서 페이지를 전환?해야 할게 생겼다.
React에서는 react-router-dom의 outlet을 이용해 쉽게 만들 수 있었는데
next에서는 어떤식으로 만드는지 궁금해졌다.
next 공식 문서를 먼저 찾아본다..
If you need multiple layouts, you can add a property getLayout to your page, allowing you to return a React component for the layout. This allows you to define the layout on a per-page basis. Since we’re returning a function, we can have complex nested layouts if desired.
여러 레이아웃이 필요한 경우, 페이지에 getLayout 속성을 추가하여 레이아웃에 대한 React 컴포넌트를 반환할 수 있습니다. 이를 통해 페이지 단위로 레이아웃을 정의할 수 있습니다. 함수를 반환하기 때문에 원하는 경우 복잡한 중첩 레이아웃을 가질 수 있습니다.
1 | import type { ReactElement } from 'react' |
1 | import type { ReactElement, ReactNode } from 'react' |
역시 공식문서는 봐도 어렵다
그냥 똑같이 만들어 본다.
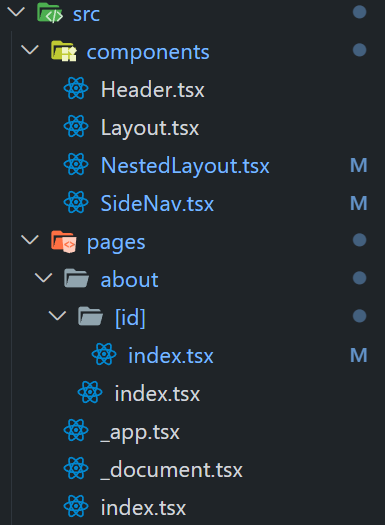
일단 파일은 이렇게 만들었다.
Header를 담고 있는 전체 Layout, 특정 페이지에만 적용될 NestedLayout
1 | import React from 'react' |
레이아웃 컴포넌트를 만들고
1 | import '@/styles/globals.css' |
페이지 컴포넌트 내에 getLayout 메서드가 있으면 그것을 사용하고, 없으면 입력으로 받은 페이지를 그대로 반환한다.
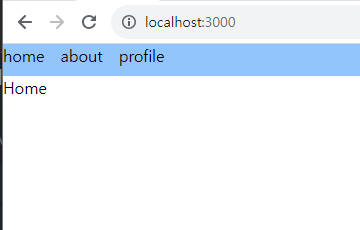
헤더가 적용된 layout
about 페이지에는 SideNav를 넣어 /about/1
, /about/2
이런식으로 라우팅을 할 것이다.
1 | import React from 'react' |
NestedLayout을 정의하고
1 | import React from 'react' |
About페이지를 NestedLayout으로 감싸준다.
getLayout 메소드가 있으므로 _app
에서 Component.getLayout
이 Return된다.
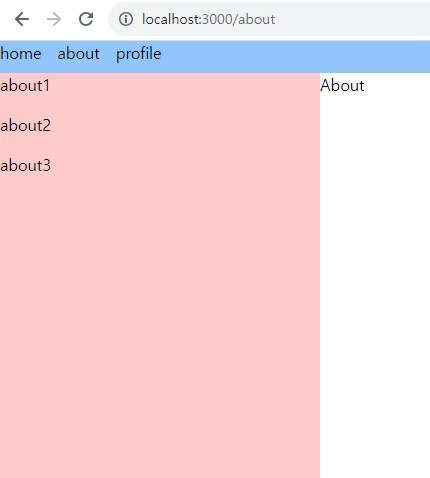